This page covers the key concepts of AG Grid: A high performance, feature rich, JavaScript Table and JavaScript Data Grid.
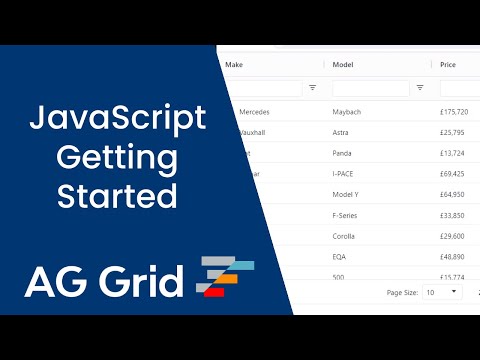
Your First JavaScript Data Grid
Add AG Grid to your application in these steps:
1. Provide a Container
Load the AG Grid library and create a blank container div:
<html lang="en">
<head>
<!-- Includes all JS & CSS for the JavaScript Data Grid -->
<script src="https://cdn.jsdelivr.net/npm/ag-grid-community/dist/ag-grid-community.min.js"></script>
</head>
<body>
<!-- Your Data Grid container -->
<div id="myGrid"></div>
</body>
</html>
2. Instantiating the JavaScript Data Grid
Create the Data Grid inside of your container div using createGrid
.
// Grid Options: Contains all of the Data Grid configurations
const gridOptions = {};
// Your Javascript code to create the Data Grid
const myGridElement = document.querySelector('#myGrid');
agGrid.createGrid(myGridElement, gridOptions);
3. Define Rows and Columns
// Grid Options: Contains all of the Data Grid configurations
const gridOptions = {
// Row Data: The data to be displayed.
rowData: [
{ make: "Tesla", model: "Model Y", price: 64950, electric: true },
{ make: "Ford", model: "F-Series", price: 33850, electric: false },
{ make: "Toyota", model: "Corolla", price: 29600, electric: false },
],
// Column Definitions: Defines the columns to be displayed.
columnDefs: [
{ field: "make" },
{ field: "model" },
{ field: "price" },
{ field: "electric" }
]
};
4. Styling the JavaScript Data Grid
Add the ag-theme-quartz
CSS class to your Data Grid container div to apply the Data Grid's theme.
<!-- Your Data Grid container -->
<div id="myGrid" class="ag-theme-quartz" style="height: 500px"></div>
5. Running the JavaScript Data Grid
Below is a live example of the application running. Click </> Code
to see the code.
To live-edit the code, open the example in CodeSandbox or Plunker using the buttons to the lower-right.
Now that you have a basic JavaScript Data Grid running, the remainder of this page explores some of the key concepts.
Showing Data
Mapping Values
The field
or valueGetter
attributes Map Data to Columns. A field maps to a field in the data. A Value Getter is a function callback that returns the cell value.
The headerName
provides the title for the header. If missing the title is derived from field
.
const gridOptions = {
columnDefs: [
{ headerName: "Make & Model", valueGetter: p => p.make + ' ' + p.model},
{ field: "price" },
],
// other grid options ...
}
Text Formatting
Format text for cell content using a Value Formatter.
const gridOptions = {
columnDefs: [
{ field: "price", valueFormatter: p => '£' + p.value.toLocaleString() },
],
// other grid options ...
}
Cell Components
Add buttons, checkboxes or images to cells with a Cell Component.
class CustomButtonComponent {
eGui;
eButton;
eventListener;
init(params) {
this.eGui = document.createElement("div");
let button = document.createElement("button");
button.className = "btn-simple";
button.textContent = "Push Me!";
this.eventListener = () => alert("clicked");
button.addEventListener("click", this.eventListener);
this.eGui.appendChild(button);
}
getGui() {
return this.eGui;
}
refresh(params) {
return true;
}
destroy() {
if (button) {
button.removeEventListener("click", this.eventListener);
}
}
}
const columnDefs = [
{ field: "button", cellRenderer: CustomButtonComponent },
// ...
];
Resizing Columns
Columns are Resized by dragging the Column Header edges. Additionally assign flex
values to allow columns to flex to the grid width.
const gridOptions = {
columnDefs: [
{ field: "make", flex: 2 }, //This column will be twice as wide as the others
{ field: "model", flex: 1 },
{ field: "price", flex: 1 },
{ field: "electric", flex: 1 }
],
// other grid options ...
}
Example
This example demonstrates mapping and formatting values, cell components, and resizing columns.
Working with Data
By default, the row data is used to infer the Cell Data Type. The cell data type allows grid features, such as filtering and editing, to work without additional configuration.
Filtering
Column Filters are embedded into each column menu. These are enabled using the filter
attribute. The filter type is inferred from the cell data type.
const gridOptions = {
columnDefs: [
{ field: "make", filter: true },
],
// other grid options ...
}
There are 5 Provided Filters which can be set through this attribute. You can also create your own Custom Filter.
Floating Filters embed the Column Filter into the header for ease of access.
const gridOptions = {
columnDefs: [
{ field: "make", filter: true, floatingFilter: true },
],
// other grid options ...
}
Editing
Enable Editing by setting the editable
attribute to true
. The cell editor is inferred from the cell data type.
const gridOptions = {
columnDefs: [
{ field: "make", editable: true },
],
// other grid options ...
}
Set the cell editor type using the cellEditor
attribute. There are 7 Provided Cell Editors which can be set through this attribute. You can also create your own Custom Editors.
const gridOptions = {
columnDefs: [
{
field: "make",
editable: true,
cellEditor: 'agSelectCellEditor',
cellEditorParams: {
values: ['Tesla', 'Ford', 'Toyota'],
},
},
],
// other grid options ...
}
Sorting
Data is Sorted by clicking the column headers. Sorting is enabled by default.
Row Selection
Row Selection is enabled using the rowSelection
attribute. Use the checkboxSelection
column definition attribute to render checkboxes for selection.
const gridOptions = {
// Column Definitions: Defines the columns to be displayed.
columnDefs: [
{ field: "make", checkboxSelection: true },
],
rowSelection: 'multiple',
// other grid options ...
}
Pagination
Enable Pagination by setting pagination
to be true.
const gridOptions = {
pagination: true,
paginationPageSize: 500,
paginationPageSizeSelector: [200, 500, 1000],
// other grid options ...
}
Example
This example demonstrates filtering, editing, sorting, row selection, and pagination.
Themes & Style
Themes
Grid Themes define how the grid looks (colors, font, spacing etc). The grid comes with Provided Themes such as Quartz and Alpine. To use a theme you need to 1) import the themes CSS and 2) apply the theme to the parent HTML element of the grid.
import "ag-grid-community/styles/ag-theme-quartz.css"; // import Quartz theme
// import "ag-grid-community/styles/ag-theme-alpine.css"; // import Alpine theme, not used here
<!-- Your grid container -->
<div id="myGrid" class="ag-theme-quartz" style="height: 500px"></div>




Customising a Theme
Customise Themes using CSS variables.
.ag-theme-quartz {
/* Changes the color of the grid text */
--ag-foreground-color: rgb(14, 68, 145);
/* Changes the color of the grid background */
--ag-background-color: rgb(241, 247, 255);
/* Changes the header color of the top row */
--ag-header-background-color: rgb(228, 237, 250);
/* Changes the hover color of the row*/
--ag-row-hover-color: rgb(216, 226, 255);
}
Figma
If you are designing within Figma, you can use the AG Grid Design System to replicate the Quartz and Alpine AG Grid themes within Figma. These default themes can be extended with Figma variables to match any existing visual design or create entirely new AG Grid themes. These can then be exported and generated into new AG Grid themes.
Cell Style
Define rules to apply Styling to Cells using cellClassRules
. This can be used, for example, to set cell background colour based on its value.
.rag-green {
background-color: #33cc3344;
}
const gridOptions = {
columnDefs: [{
field: 'electric',
cellClassRules: {
// apply green to electric cars
'rag-green': params => params.value === true,
}
}],
// other grid options ...
}
Row Style
Define rules to apply Styling to Rows using rowClassRules
. This allows changing style (e.g. row colour) based on row values.
.rag-red {
background-color: #cc222244;
}
const gridOptions = {
rowClassRules: {
// apply red to Ford cars
'rag-red': params => params.data.make === 'Ford',
},
// other grid options ...
}
Example
This example demonstrates cell style and row style.
Next Steps
- Read our Introductory Tutorial.
- Watch our Video Tutorials.